Diskselector¶
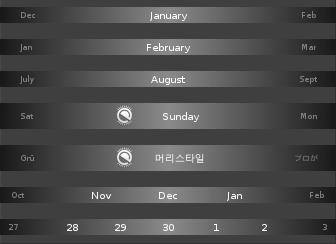
Widget description¶
A diskselector is a kind of list widget. It scrolls horizontally, and can contain label and icon objects. Three items are displayed with the selected one in the middle.
It can act like a circular list with round mode and labels can be reduced for a defined length for side items.
Emitted signals¶
selected
- when item is selected, i.e. scroller stops.clicked
- This is called when a user clicks an itemscroll,anim,start
- scrolling animation has startedscroll,anim,stop
- scrolling animation has stoppedscroll,drag,start
- dragging the diskselector has startedscroll,drag,stop
- dragging the diskselector has stopped
Note
The scroll,anim,*
and scroll,drag,*
signals are only emitted
by user intervention.
Layout content parts¶
icon
- An icon in the diskselector item
Layout text parts¶
default
- Label of the diskselector item
Scrollable Interface¶
This widget supports the scrollable interface.
If you wish to control the scrolling behaviour using these functions,
inherit both the widget class and the
Scrollable
class
using multiple inheritance, for example:
class ScrollableGenlist(Genlist, Scrollable):
def __init__(self, canvas, *args, **kwargs):
Genlist.__init__(self, canvas)
Inheritance diagram¶

- class efl.elementary.Diskselector(Object parent, *args, **kwargs)¶
Bases:
efl.elementary.__init__.Object
This is the class that actually implements the widget.
- Parameters
parent (
efl.evas.Object
) – The parent object**kwargs – All the remaining keyword arguments are interpreted as properties of the instance
- bounce¶
Deprecated since version 1.8: You should combine with Scrollable class instead.
- bounce_get()¶
Deprecated since version 1.8: You should combine with Scrollable class instead.
- bounce_set(h, v)¶
Deprecated since version 1.8: You should combine with Scrollable class instead.
- callback_clicked_add(func, *args, **kwargs)¶
This is called when a user clicks an item
New in version 1.8.
- callback_clicked_del(func)¶
- callback_scroll_anim_start_add(func, *args, **kwargs)¶
Scrolling animation has started.
- callback_scroll_anim_start_del(func)¶
- callback_scroll_anim_stop_add(func, *args, **kwargs)¶
Scrolling animation has stopped.
- callback_scroll_anim_stop_del(func)¶
- callback_scroll_drag_start_add(func, *args, **kwargs)¶
Dragging the diskselector has started.
- callback_scroll_drag_start_del(func)¶
- callback_scroll_drag_stop_add(func, *args, **kwargs)¶
Dragging the diskselector has stopped.
- callback_scroll_drag_stop_del(func)¶
- callback_selected_add(func, *args, **kwargs)¶
When item is selected, i.e. scroller stops.
- callback_selected_del(func)¶
- clear()¶
Remove all diskselector’s items.
See also
delete()
item_append()
- display_item_num¶
The number of items to be displayed.
Default value is 3, and also it’s the minimum. If
num
is less than 3, it will be set to 3.Also, it can be set on theme, using data item
display_item_num
on group “elm/diskselector/item/X”, where X is style set. E.g.:group { name: "elm/diskselector/item/X"; data { item: "display_item_num" "5"; }
- Type
int
- first_item¶
Get the first item of the diskselector.
The list of items follows append order. So it will return the first item appended to the widget that wasn’t deleted.
See also
- Type
- item_append(label, icon=None, callback=None, *args, **kwargs)¶
A constructor for
DiskselectorItem
- items¶
Get a list of all the diskselector items.
See also
item_append()
delete()
clear()
- Type
list of
DiskselectorItem
- last_item¶
Get the last item of the diskselector.
The list of items follows append order. So it will return last first item appended to the widget that wasn’t deleted.
See also
- Type
- round_enabled¶
Enable or disable round mode.
Disabled by default. If round mode is enabled the items list will work like a circular list, so when the user reaches the last item, the first one will popup.
- Type
bool
- scroller_policy¶
Deprecated since version 1.8: You should combine with Scrollable class instead.
- scroller_policy_get()¶
Deprecated since version 1.8: You should combine with Scrollable class instead.
- scroller_policy_set(policy_h, policy_v)¶
Deprecated since version 1.8: You should combine with Scrollable class instead.
- selected_item¶
Get the selected item.
The selected item can be unselected with function
DiskselectorItem.selected
, and the first item of diskselector will be selected.The selected item always will be centered on diskselector, with full label displayed, i.e., max length set to side labels won’t apply on the selected item. More details on
side_text_max_length
.- Type
- side_text_max_length¶
The side labels max length.
Length is the number of characters of items’ label that will be visible when it’s set on side positions. It will just crop the string after defined size. E.g.:
An item with label “January” would be displayed on side position as “Jan” if max length is set to 3, or “Janu”, if this property is set to 4.
When it’s selected, the entire label will be displayed, except for width restrictions. In this case label will be cropped and “…” will be concatenated.
Default side label max length is 3.
This property will be applied over all items, included before or later this function call.
- Type
int
- class efl.elementary.DiskselectorItem(label=None, Object icon=None, callback=None, cb_data=None, *args, **kargs)¶
Bases:
efl.elementary.__init__.ObjectItem
An item for the
Diskselector
widget.A new item will be created and appended to the diskselector, i.e., will be set as last item. Also, if there is no selected item, it will be selected. This will always happens for the first appended item.
If no icon is set, label will be centered on item position, otherwise the icon will be placed at left of the label, that will be shifted to the right.
Items created with this method can be deleted with
delete()
.If a function is passed as argument, it will be called every time this item is selected, i.e., the user stops the diskselector with this item on center position.
Simple example (with no function callback or data associated):
disk = Diskselector(win) ic = Icon(win, file="path/to/image", resizable=(True, True)) disk.item_append("label", ic)
See also
delete()
Diskselector.clear()
Image
- Parameters
label (string) – The label of the diskselector item.
icon (
Object
) – The icon object to use at left side of the item. An icon can be any Evas object, but usually it is anIcon
.callback (callable) – The function to call when the item is selected.
cb_data – User data for the callback function
**kwargs – All the remaining keyword arguments are interpreted as properties of the instance
- append_to(diskselector)¶
Appends a new item to the diskselector object.
- Returns
The created item or
None
upon failure.- Return type
- next¶
Get the item after
item
in diskselector.The list of items follows append order. So it will return item appended just after
item
and that wasn’t deleted.If it is the last item,
None
will be returned. Last item can be get byDiskselector.last_item
.- Type
- prev¶
Get the item before
item
in diskselector.The list of items follows append order. So it will return item appended just before
item
and that wasn’t deleted.If it is the first item,
None
will be returned. First item can be get byDiskselector.first_item
.- Type
- selected¶
The selected state of an item.
This sets the selected state of the given item.
True
for selected,False
for not selected.If a new item is selected the previously selected will be unselected. Previously selected item can be fetched from the property
Diskselector.selected_item
.If the item is unselected, the first item of diskselector will be selected.
Selected items will be visible on center position of diskselector. So if it was on another position before selected, or was invisible, diskselector will animate items until the selected item reaches center position.
See also
- Type
bool